SPiCboard
Serial communication with SPiCboards. More...
Files | |
file | com.h |
Macros | |
#define | BAUD_RATE |
#define | sb_com_wait(FACTOR) |
Enumerations | |
enum | COM_ERROR_STATUS { ERROR_NO_STOP_BIT , ERROR_PARITY , ERROR_BUFFER_FULL , ERROR_INVALID_POINTER } |
Error states used in mask. More... | |
Functions | |
void | sb_com_sendByte (uint8_t data) |
Send a single byte (8-bit) plus one start-, parity and stop bit. More... | |
uint8_t | sb_com_receiveByte (uint8_t *data) |
Receive one byte with start, parity and stop bit each. More... | |
Detailed Description
Serial communication with SPiCboards.
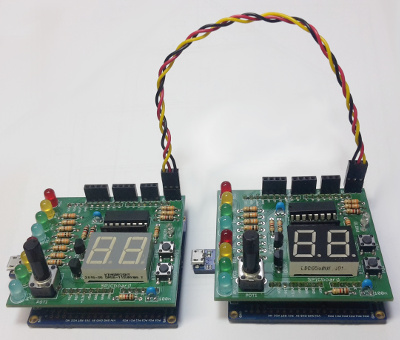
The SPiCboard3 can perform serial communication using the three PINs on its upper right corner (TX
at PD1
, GND
and RX
at PD0
). To establish a connection you have to connect its RX
with TX
of another board, both GND
pins and TX
with RX
, like shown on the image above.
For the transmission this library uses the transmission setting 8-E-1
, in which we send (in addition to the start bit) 8 data bits, one even parity bit and one stop bit.
Example: Transmission of the (decimal) value 23
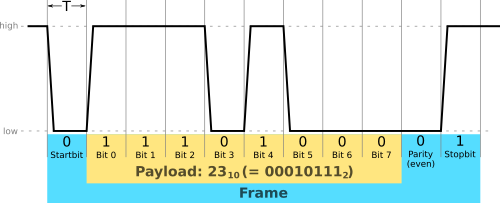
PD0
is configured as input (RX
)PD1
is configured as output (TX
)- The idle voltage is 5V, a logical high (this is a historic legacy from telegraphy, in which the line is held high to show that the line and transmitter are not damaged).
- the transmission begins with the start bit, which changes the level from logical high (idle) to logical low.
- the time interval
T
for transmitting one symbol is defined by theBAUD_RATE
: - the payload byte is sent bitwise, starting with the least significant bit
- the parity bit ensures that an even number of logical highs has been sent (including parity - calculated using
XOR
) - it can be used to detect transmission errors. - the stopbit finishes the transmission and leaves the line in a logical high
- Note
- The libspicboard implementation uses the 16bit timer 4.
- For educational purposes the transmission is implemented completely in software - for more advanced serial communcation you can use USART, described in Section 25 of the ATMega328PB Datasheet.
- See also
- Extended Serial Communication
Macro Definition Documentation
#define BAUD_RATE |
Default baud rate (symbols per second) is 1200
#define sb_com_wait | ( | FACTOR | ) |
Active wait loop for the transmission interval time T
(determined by the baud rate) multiplied by FACTOR
Enumeration Type Documentation
enum COM_ERROR_STATUS |
Function Documentation
uint8_t sb_com_receiveByte | ( | uint8_t * | data | ) |
Receive one byte with start, parity and stop bit each.
We try to sample in the middle of each symbol, therefore we have to wait about after receiving the start bit before reading the first data bit. Every subsequent symbol is sampled after a
delay.
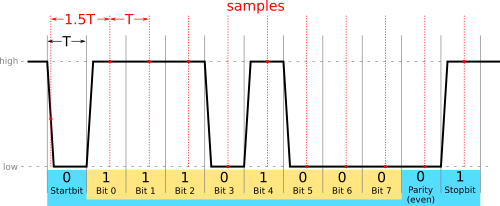
Transmission errors are recorded and returned. They should not be ignored, because they usually indicate invalid data - there is no resend mechanism implemented in this library!
- Parameters
-
data pointer to allocated space for the received byte
- Return values
-
0 no error >0 bit mask describing the error: - 1. bit: no stop bit received (
ERROR_NO_STOP_BIT
) - 2. bit: parity bit wrong (
ERROR_PARITY
) - 3. bit: receive buffer full (
ERROR_BUFFER_FULL
) - 4. bit: data pointer invalid (
ERROR_INVALID_POINTER
)
- 1. bit: no stop bit received (
void sb_com_sendByte | ( | uint8_t | data | ) |
Send a single byte (8-bit) plus one start-, parity and stop bit.
- Note
- Shall not be called inside a ISR!
- Parameters
-
data the byte to send